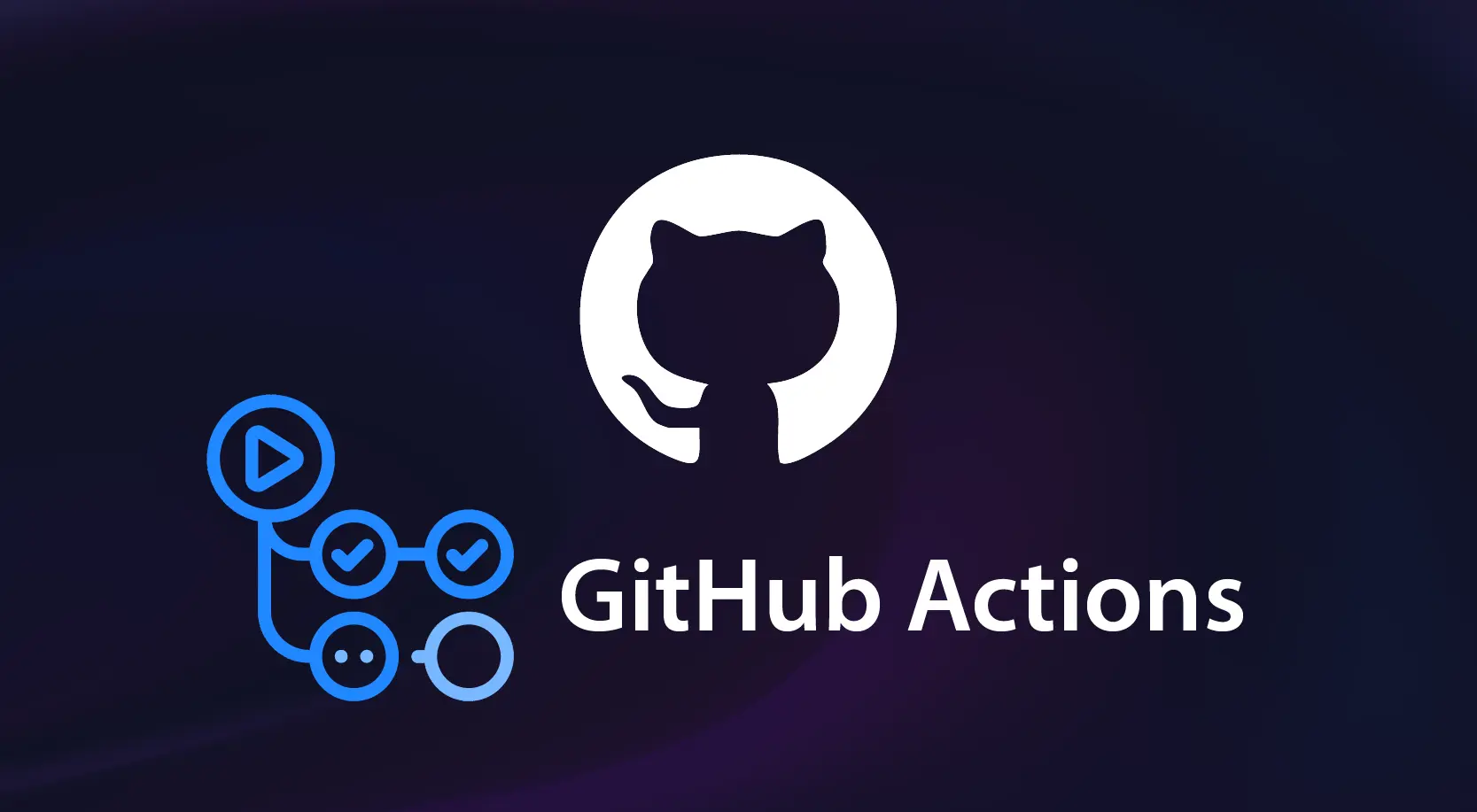
GitHub has become a core tool for developers around the world, helping them manage and collaborate on projects of all sizes. One of the most powerful features GitHub offers is GitHub Actions, which allows you to automate, customize, and execute your software development workflows directly from your GitHub repository. It enables continuous integration and continuous deployment (CI/CD), allowing you to build, test, and deploy your code automatically whenever you push changes.
Key Features of GitHub Actions
- Workflows: Defined in YAML files, workflows specify a series of tasks (jobs) that run in response to events like code pushes, pull requests, or on a schedule.
- Jobs: A workflow can contain multiple jobs, which run in parallel or sequentially. Each job can execute commands, run scripts, or use actions.
- Actions: Reusable units of code that perform specific tasks. You can create your own actions or use community-contributed ones from the GitHub Marketplace.
- Triggers: Workflows can be triggered by various GitHub events, such as pushes, pull requests, releases, or even on a schedule.
- Secrets: Securely store sensitive information (like API keys or passwords) to be used in your workflows without exposing them in your code.
- Matrix Builds: Run your jobs in different environments or configurations simultaneously (e.g., different versions of a language or OS).
Benefits of Using GitHub Actions
- Increased Efficiency/ Automation: Automate repetitive tasks like testing and deployment, saving time and reducing errors. GitHub Actions allows you to automate repetitive tasks, reducing manual effort and minimizing errors. By automating such tasks as code testing and deployment, you can focus more on writing code and less on managing the development process.
- Seamless Integration: Seamlessly integrates with your GitHub repositories, making it easy to implement CI/CD. GitHub Actions seamlessly integrates with your GitHub repositories, enabling you to define workflows directly within your code base on GitHub. This tight integration ensures that your workflows are version controlled and easily accessible to your team with all of the other great features that GitHub offers.
- Customizability: Highly customizable workflows to fit your specific project needs. With GitHub Actions, you have the flexibility to define custom workflows tailored to your specific requirements. So, whether you’re building a simple CI/CD pipeline or orchestrating complex, multi‑step workflows, GitHub Actions can help you automate all of them.
- Community: A large community and marketplace for actions, making it easy to find pre-built solutions.
- Cost: Another benefit is that GitHub Actions are relatively cost‑effective. GitHub Actions provide generous free usage limits for all public repositories and includes a certain number of free minutes for private repositories. GitHub plans also offer free usage up to a certain limit depending on your plan choice. This makes it a cost‑effective option for teams and projects of all sizes, especially if you’re already using GitHub.
Use Cases – GitHub Actions
- Continuous Integration: Automatically run tests every time code is pushed to ensure code quality. Continuous integration is a software development practice where code changes are automatically built, tested, and integrated into the main code base frequently. GitHub Actions makes implementing CI pipelines seamlessly by allowing you to define workflows that automatically trigger on such events like a create, a push, or a schedule just to name a few.
- Deployment: Automatically deploy code to a server or cloud provider when changes are merged into the main branch.
- Notification: Send notifications (e.g., Slack messages) when specific events occur in your repository. We use it to notify team members or external systems when specific events occur, such as the creation of a new issue, a pull request, review request, or a deployment status update. We also use GitHub Actions on a schedule to send custom notifications to Slack, Microsoft Teams, and other communication channels to keep the team informed about weekly standup discussions and other team‑related information.
- Automation: you can automate deployment and deploy your application to various environments, such as staging or production, based on predefined conditions or those event triggers. You can implement rollback mechanisms to revert the previous versions in case of deployment failures or issues.
Some other common use cases include issue management, pull request automation, repository maintenance, and community engagement.
How to create a GitHub Actions workflow
Before we dive in and create a basic workflow file, let’s first understand the components within that file. A GitHub Actions workflow file is written in YAML and consists of several key components that define how the workflow operates. These workflows follow a specific structure and are stored in the
.github/workflows directory of your GitHub repository.
Here’s an overview of the main components:
So, let’s break down the main components of a workflow file. The first component we see is name.
1. Name
This is an optional field that provides a name for the workflow. The name component is a descriptive title for your workflow and can really be anything that you want. It’s a way to give your workflow a recognizable identity, making it easier for you and others to understand its purpose.
Example:
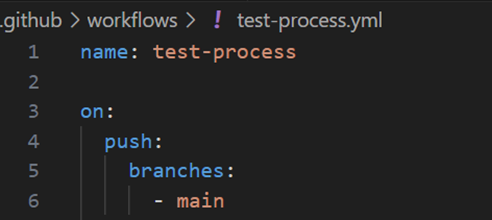
2. on:
Specifies the events that trigger the workflow, such as pushes, pull requests, or scheduled events. It’s like setting up the conditions under which your workflow should run. And you can choose from a variety of events, such as pushes to the repository, pull requests, issue comments, and much more.
Example:
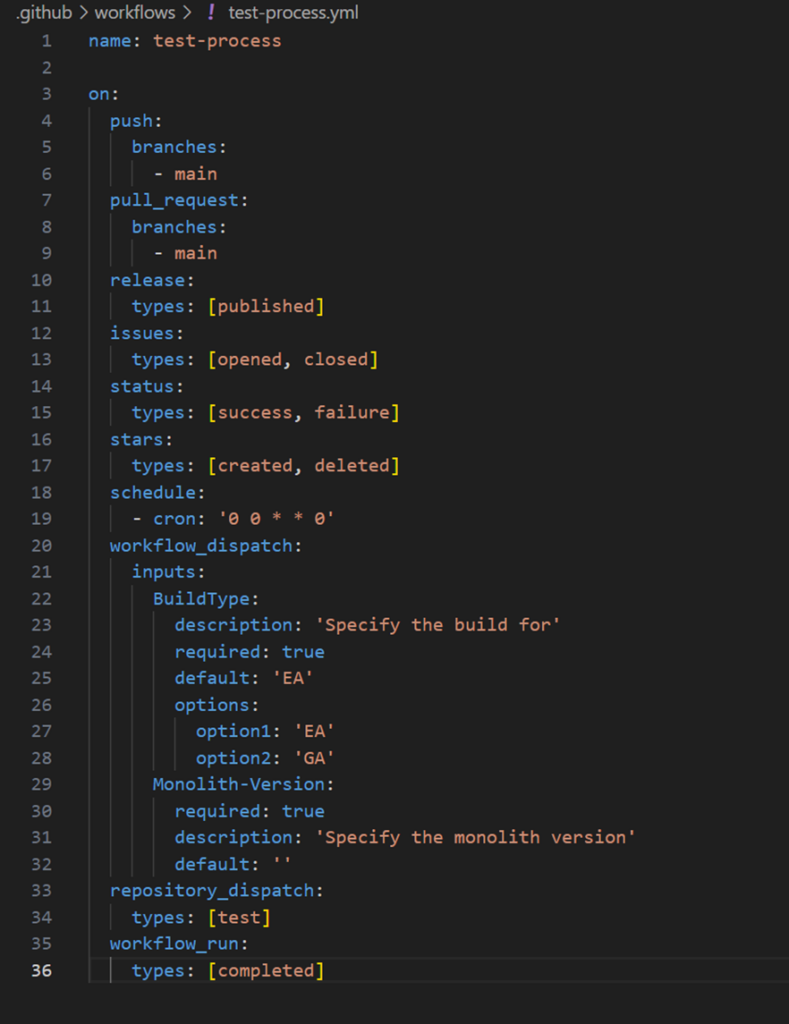
These events correspond to actions that occur in your repository:
- push: Triggers when code is pushed to the repository.
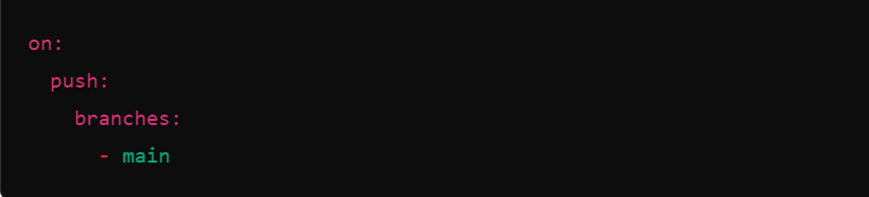
ii. pull_request: Triggers when a pull request is opened, synchronized, or reopened.
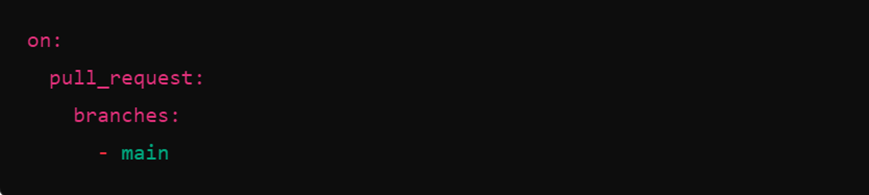
iii. release: Triggers when a release is published or created.

iv. issues: Triggers on issue events (e.g., opened, closed).

v. fork: Triggers when someone forks the repository.

vi. star: Triggers when someone stars the repository.

vii. Schedule Events: You can set up workflows to run on a schedule using cron syntax. This event will only trigger a workflow run if the workflow file is on the default branch. Scheduled workflows will only run on the default branch.

Cron syntax has five fields separated by a space, and each field represents a unit of time.
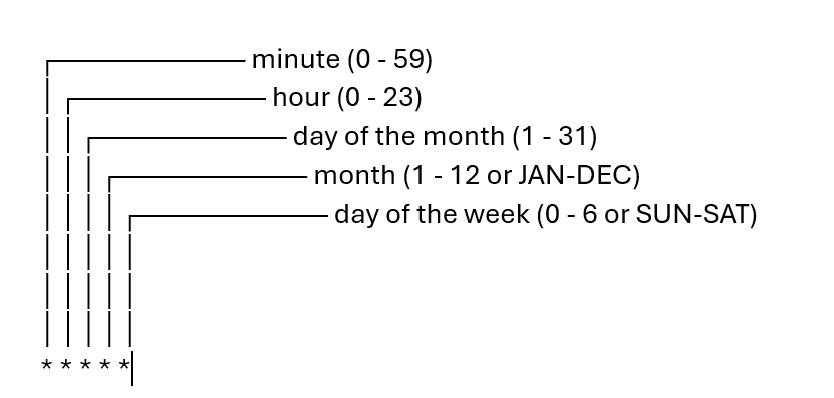
You can use these operators in any of the five fields:
Operator | Description | Example |
* | Any value | 15 * * * * runs at every minute 15 of every hour of every day. |
, | Value list separator | 2,10 4,5 * * * runs at minute 2 and 10 of the 4th and 5th hour of every day. |
– | Range of values | 30 4-6 * * * runs at minute 30 of the 4th, 5th, and 6th hour. |
/ | Step values | 20/15 * * * * runs every 15 minutes starting from minute 20 through 59 (minutes 20, 35, and 50). |
viii. Workflow Dispatch: It allows you to manually trigger the workflow from the GitHub UI. When using the workflow_dispatch event, you can optionally specify inputs that are passed to the workflow. This trigger only receives events when the workflow file is on the default branch. The triggered workflow receives the inputs in the inputs context (line 5). The maximum number of top-level properties for inputs is 10.
Example:
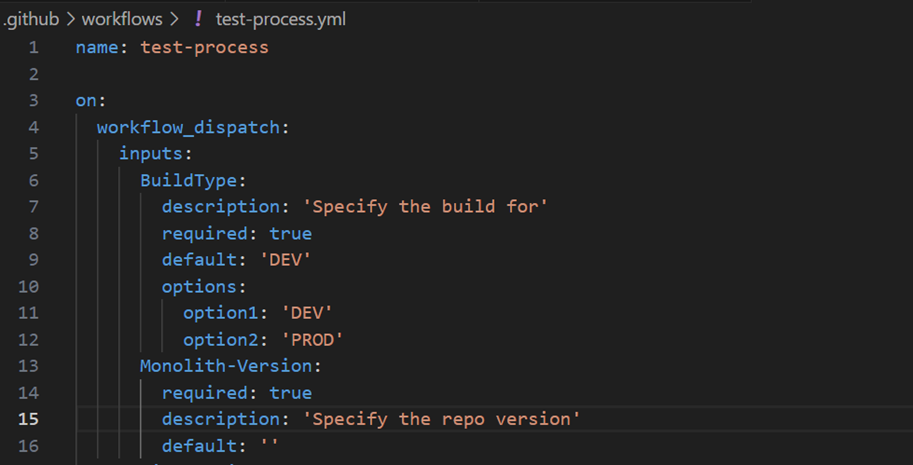
ix. Repository Dispatch: repository_dispatch is a GitHub Actions event that allows you to trigger workflows from external sources or other GitHub repositories. This event can be used to send custom payloads and trigger workflows based on specific conditions.
- Setup: You define a workflow in your repository that listens for the repository_dispatch event. This is done in your workflow YAML file:

- Triggering the Event: To trigger the event, you can use the GitHub API. You need to make a POST request to the GitHub API endpoint, including a custom event type and any additional data you want to send in the payload.
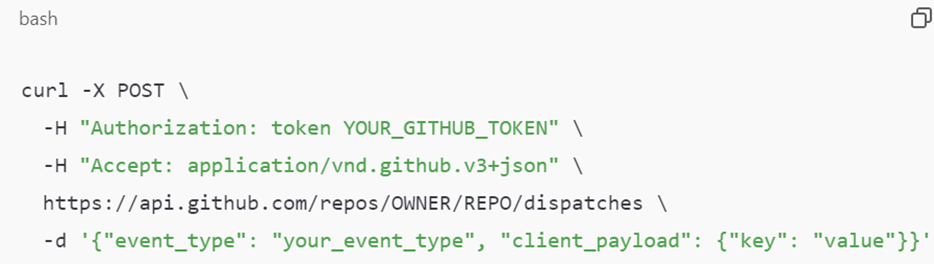
- Handling the Event: Inside your workflow, you can access the client_payload to utilize the data sent with the event. This allows you to customize the behavior of your workflow based on the information received.
x. Filtering: You can further refine the triggering events using filters for branches, tags, and paths. For example:
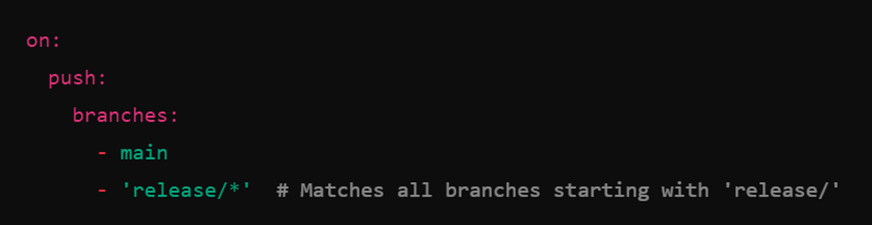

3. jobs
- Description: Contains a collection of jobs that will run as part of the workflow. Each job runs in its own environment.

4. steps
- Description: A job consists of multiple steps, which can run commands, call actions, or run scripts.
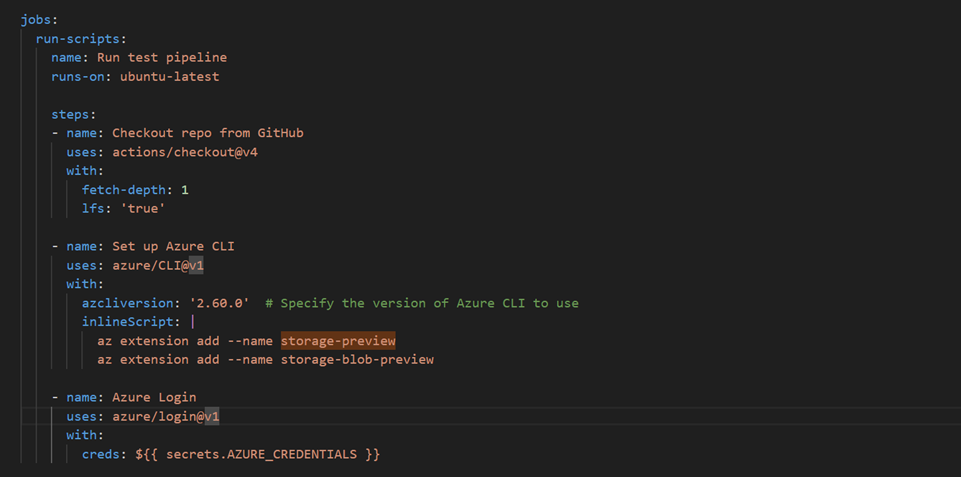
- Uses: Indicates the action to be used in a step. Actions can be GitHub-hosted or custom.
- Run: Specifies a command or script to execute in a step.
5. env
- Description: Define environment variables that can be used in steps.
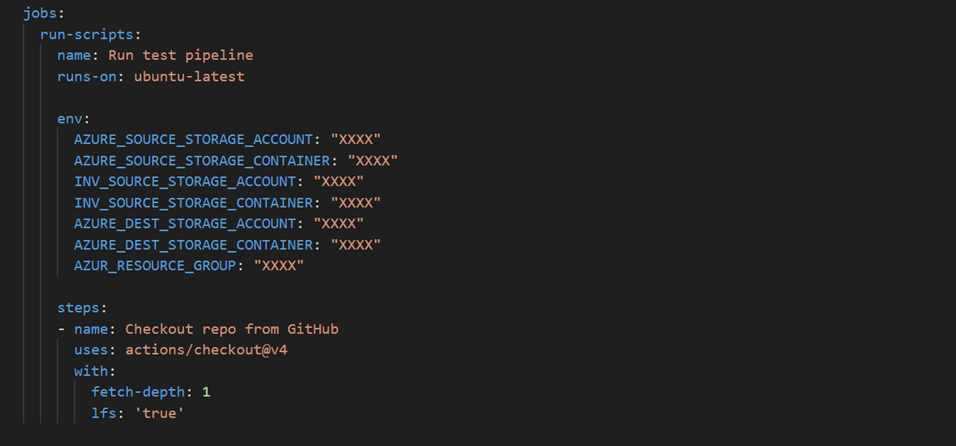
6. secrets
- Description: Allows access to secrets stored in the repository settings, used for sensitive data like API keys.

7. outputs
- Description: Define outputs from a job that can be used in subsequent jobs. In GitHub Actions, output refers to the data that a step or job produces and can be used in subsequent steps or jobs within a workflow. Outputs allow you to pass information between different parts of your workflow.
- Defining Outputs: You can define outputs in a job using the echo command in the shell, or in a step using the id property and outputs section.
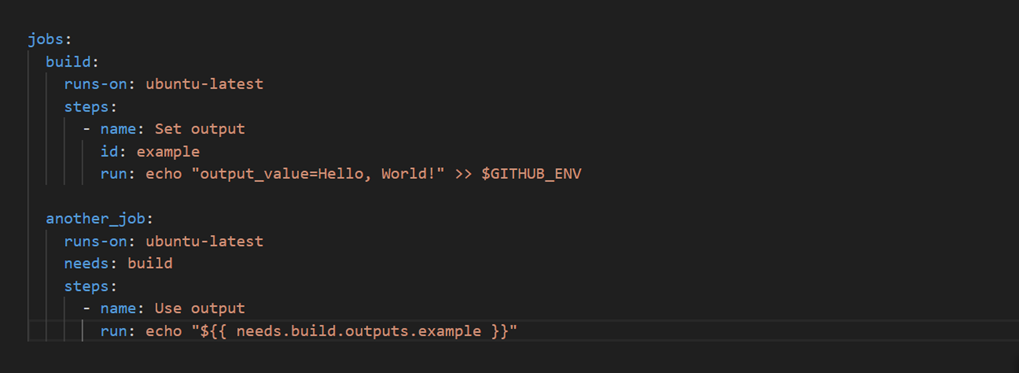
- Accessing Outputs: You can access outputs using the ${{ needs. <job_id>.outputs.<output_id> }} syntax, where <job_id> is the ID of the job that produced the output, and <output_id> is the ID of the output.
- Job-level vs. Step-level Outputs: Outputs can be defined at both job and step levels. Job-level outputs can be shared with other jobs, while step-level outputs are accessible only within the same job.
- Using in Conditions: Outputs can also be used in conditional statements to determine whether a job or step should run.
- Environment Variables: You can also set environment variables during a job, which can be used in subsequent steps but are not the same as outputs.
Some more example of Job Outputs:
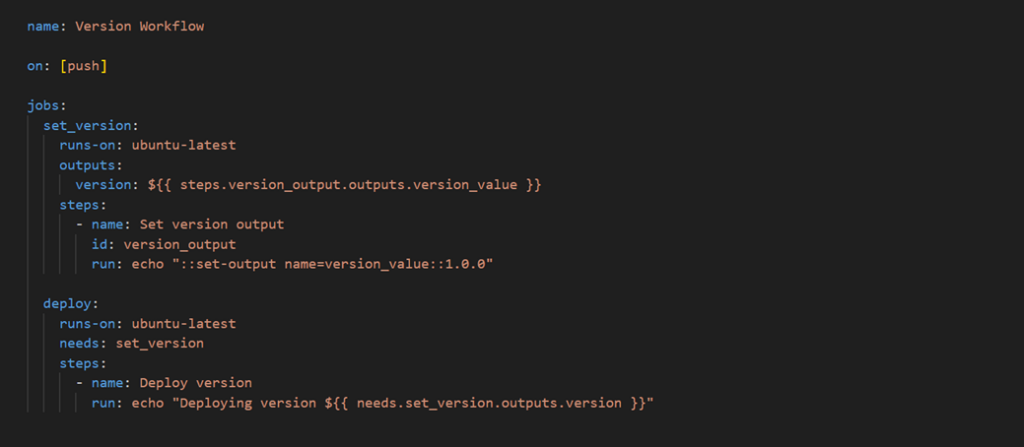
8. needs
- Description: Specifies dependencies between jobs, ensuring that a job runs only after the specified jobs have completed successfully. needs is a keyword used to define dependencies between jobs in a workflow. It ensures that a job only runs after its specified predecessor jobs have completed successfully. This is particularly useful for organizing workflows where certain tasks depend on the successful completion of others.
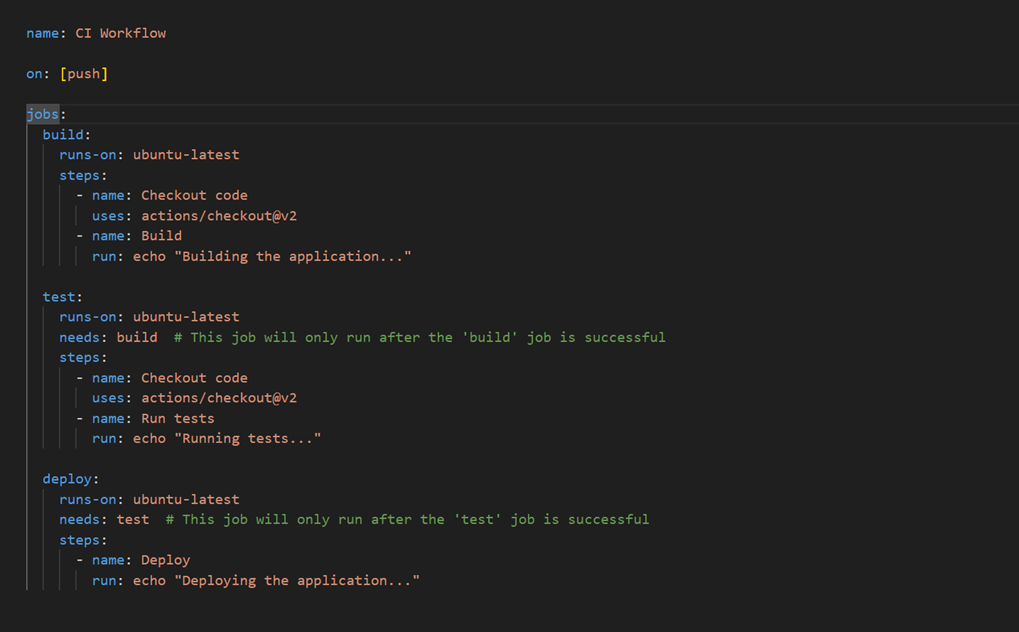
Example Workflow File
Here’s a simple example of a complete workflow file:
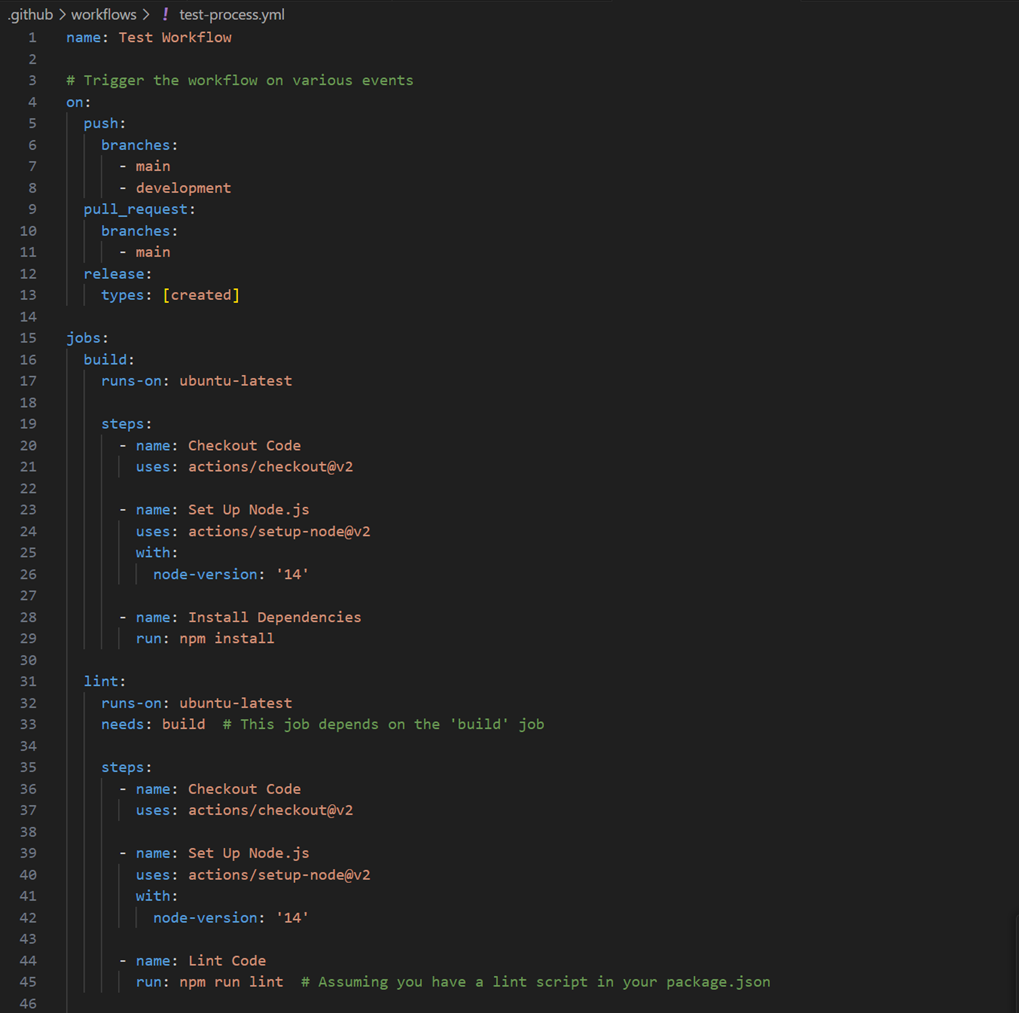
Conclusion
In summary, GitHub Actions enables you to focus on your core priorities: creating and innovating. By automating critical stages of the software lifecycle—from integration through deployment—GitHub Actions not only conserves time but also reduces the likelihood of mistakes.
Whether you’re an individual developer or part of a larger team, its adaptability and seamless integration with GitHub make it indispensable for contemporary software development.
Try it out and experience how much more efficient your workflows can be!